Daktela V6 API: developing a custom agent interface
Using the Daktela V6 API (application programming interface), you can integrate Daktela system functionality into your app or widget. The Daktela V6 API uses the REST standard and communicates using the HTTP method. Data is serialised in JSON or JSONP formats (more info in our API documentation). JSON serialisation is used in this manual.
Access Token
To communicate using the Daktela V6 API, you first need to get an access token (string) that authorises the user request. You can find the access token in the user's details. Alternatively, you can find it using an API request when logging in (see below). The access token is a mandatory part of all requests (except logging in) and has to be in the format:
/api/v6/endpoint?accessToken={MY_ACCESS_TOKEN}
A request without a valid access token will return a 401 Unauthorized response.
Pull Data
Accessing the endpoint /api/v6/appPullData, you can use long polling to detect any events. Apart from the data, the response also contains the hash attribute which identifies the user's current state. The first request is sent with the hash attribute empty. The server immediately returns all data and the hash. For long polling to work correctly, each subsequent request must contain the hash returned in the previous pull data request's response. If an event takes place, the server will immediately send a response again containing the hash as well as the data of the given event. If no event occurs, after 15 seconds the server will send a response containing the hash only (it must again be contained in the next pull data request). Pull data is an integral part of the real time application – it detects activities and their events (e.g. incoming calls), device state changes, beginnings and ends of pauses, queue logins and logouts, notifications etc.
The pull data response only contains all data after the first request with the hash attribute empty. All subsequent requests containing a valid hash return only the data (JSON keys and their values – objects or arrays) where changes have occurred. The following diagram describes an event where an incoming call starts ringing. The pull data response contains only the activities (the incoming activity) and extension (info about the user currently logged in their state) keys.
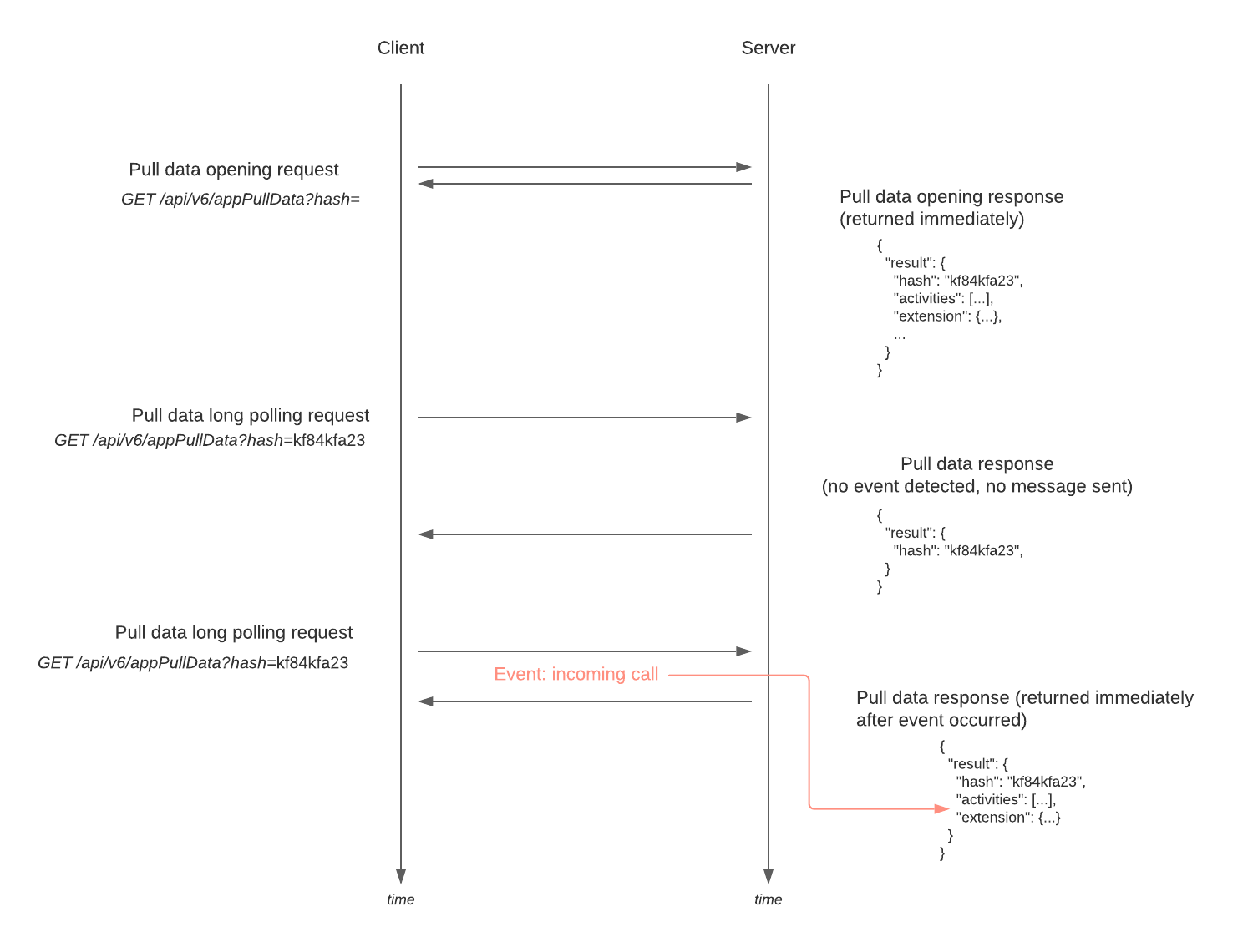
The pull data structure is as follows:
activities – open/waiting activities,
extension – info about the user and their state,
queues – the queues the user is logged in to,
missedChannels – the number of missed activities,
announcements – notifications,
reloadUser – a switch notifying that it is necessary to reload the static WhoIm data (see below).
WhoIm
The endpoint /api/v6/whoim returns static data about the user currently logged in (according to the access token). Requests are made using the HTTP GET method. If the access token is invalid, the endpoint only returns info about the current version of the Daktela V6 system.
WhoIm structure:
Log in and Out
The endpoint /api/v6/login is used to log in. It is the only endpoint that does not require an access token. It is currently not possible to log in via the API using third party OAuth (e.g. Google). To log in, an HTTP POST request needs to be sent to the endpoint with the payload
{“username”: “testuser123”, “password”: “SecretPassword”}
Username (string) contains the user identifier (name), password (string) contains the password. The user's access token will be a part of the response and may contain other data depending on the parameters used. If you add the only_token attribute (boolean) with the true value to the payload, the API will return only the access token and a cookie will not be set. After you have successfully logged in, it is recommended to start long polling to the pull data endpoint where you can access all important data.
To log out, make an HTTP GET request to the endpoint /api/v6/logout.
Go Ready/Go Unready
Unless the user has static login turned on, they must Go ready (switch to the Idle state) after logging in. This information is contained in the state attribute (string) of the extension object in pull data. If state = Session, it is necessary to Go ready.
The endpoint /api/v6/usersSession is used to Go ready. Send an HTTP POST request with the payload
{“setReady”: true}
The response will return 200 OK. If the user's device is dynamic, you must also send the number (name) of the device the user wants to connect to. E.g.
{“setReady”: true, “extension”: “305”, model: "sipDevices"}
or
{“setReady”: true, “extension”: “721322422”, model: "externalNumbers"}
The following endpoint returns a list of available devices:
/api/v6/getAvailableExtensions?onlyCC=true
To Go unready (switch to the Session state), again send an HTTP POST request to the endpoint /api/v6/usersSession, this time with the payload
{“setReady”: false}
Examples from the Daktela web app:
state = Session
state = Idle
Add/Remove Device
To add or remove a device, use the endpoint /api/v6/usersSession. An HTTP POST request with the payload
{“extension”: “310”}
logs the user in to the device 310.
An HTTP POST request with the payload
{“extension”: {“name”: “310”, “logout”: true}
logs the user out of the same device.
Start and end a Pause
When state (string) in the extension object in pull data is set to the value Paused, the user is on a pause. If this is the case, the attributes id_pause (object? – the pause they are on) and on_pause (datetime? – the date and time when the pause was started) will also be set in the extension object in pull data. If the user is not on a pause, these attributes will be null.
You can start a pause using an HTTP POST request to the endpoint /api/v6/usersSession with the payload
{“pause”: “mypause”}
where "mypause" is the pause identifier (name).
You can end a pause in a similar way. Simply edit the payload to
{“pause”: null}
Examples from the Daktela web app:
state = Idle
state = Paused
Active/Inactive Device
You can find out if the user has a reachable device from the exten_status attribute (string) of the extension object in the pull data response. When the device is reachable, exten_status will be Idle, when it is unreachable, it will be Unavailable.
Examples from the Daktela web app:
exten_status = Idle
exten_status = Unavailable
Log in and Out of Queues
You can log in and out of queues using the endpoint /api/v6/usersSession. An HTTP POST request with the payload
{“queues”: [{“name”: “1234”, “login”: true, “penalty”: “0”}]}
logs the user in to the queue with the name 1234.
An HTTP POST request with the payload
{“queues”: [{“name”: “1234”, “login”: false}]}
logs the user out of the same queue.
You can load a list of available queues at the WhoIm endpoint or from the user profile. To load the list from the user profile, send an HTTP GET request to the endpoint /api/v6/profiles/{name}/queues (where name is the user profile identifier (name)). You can get the user profile identifier from the user object stored e.g. in the id_agent attribute of the extension object in pull data.
From the loaded queues, you then need to filter those that are active and those that the user can log in to. Use the attributes deactivated (boolean) a _rdata (object) from the queue object. Deactivated will tell you if the queue has been deactivated or not. The _rdata object contains (among others) the login attribute (string) – if its value is empty, the user cannot log in to the queue (the other possible values are auto, manual and fix).
Incoming Activity
As outlined above, you can pick up any PBX events using pull data long polling. This includes an incoming activity – an incoming call in this example. When there is an incoming activity, you will find it in the activities array of the pull data response. The activity object contains numerous attributes including:
type (string) – activity type (call, email, chat etc.)
action (string) – current activity state (waiting, open, postponed, closed),
item (object?) – object with the given activity type data (see ActivitiesCall, ActivitiesEmail, ActivitiesWeb, ActivitiesSms, ActivitiesFbm, ActivitiesWap, ActivitiesVbr), (note: nullable),
user (object?) – the user that has been assigned the activity (or null if the activity has not been assigned to a user).
When an incoming activity in being distributed (or when there is no user to whom the activity can be distributed), the action attribute is empty (ie. action = ""). The activity is ringing at the current user when action = WAIT and user = currently logged in user. When the action attribute is empty of WAIT and the user attribute is null, the incoming activity is not ringing at the user (it is still however possible to accept it from waiting activities – the bell).
Examples from the Daktela web app:
action = WAIT and user = currentUser.
action = “” or (action = “WAIT” and user = null)
You can accept and incoming activity using an HTTP PUT request to given activity's endpoint (/api/v6/activities/{name} where name is the activity identifier) with the payload
{“action”: “OPEN”, “_exten”: “305”}
_exten (string) determines the device (its identifier – name) that will handle the activity. If the user has exactly one device or if the activity type is not a call (e.g. a chat), you can leave out the _exten attribute from the payload. If successful, the server will return a 200 OK response as well as the response to the pull data polling request that will now contain action = OPEN in the given activity.
You can reject an incoming activity in a similar way – an HTTP PUT request to the endpoint /api/v6/activities/{name} with the payload
{“reject”: true}
Create an Outgoing Call Activity
To dial a call, send an HTTP POST request to the endpoint /api/v6/activities with the payload
{“type“: “CALL“, “number“: “111222333“}
where number is the number you want to dial. The user has to be logged in to an outgoing call queue.
End a Aall and Close an Activity
You can find out the call state in call channels. The activity object returned in pull data contains the item object (see above) where the given activity type data is stored. If the activity is a call, the ActivitiesCall object will be stored in the item attribute. A list of call channels is stored in the channels array of this object (note: nullable). The current user's call channel has the current user's object stored in the user attribute (ie. it is a channel where user = currentUser). If the call channel's state attribute has a different value than Closed, the call is ongoing. To end the call, send an HTTP PUT request to the endpoint /api/v6/activities/{name} with the payload
{“hangup”: {“channel“: null}}
where the channel attribute (string?) is the channel that should be ended – if its value is null, the current user's channel will be ended.
You can close an activity (any type, not just a call) using an HTTP PUT request to the endpoint /api/v6/activities/{name} with the payload
{“action”: “CLOSE“}
If the activity you are closing is in a queue which has statuses, you need to include them in your payload as an array with individual status identifiers (name attribute). E.g. the payload
{“action”: “CLOSE“, “statuses“: [“status1”, “status2”]}
sent in a request to the endpoint stated above closes the activity and sets its statuses (with identifiers (name) status1 and status2).
Work With Errors Returned By the Daktela V6 API
If you send an invalid request, the Daktela API will return a 400 Bad Request response. The body of the response contains the error attribute as standard. If the response is a status code 2xx, the error attribute is set to null. On the other hand the if response is a status code 400, the error attribute will contain the error message (localised according to the Accept-Language header). E.g. if you are trying to remove a device (see above – HTTP post request to the endpoint /api/v6/usersSession) from a user who is logged in to a call queue, a response with a status code 400 will be returned with the following body
{"result": false,"error": ["User is logged in to queues which require at least one device"]}
This way you can show error messages returned by the Daktela V6 API to users.
The error attribute can contain an array of strings or an object. The error object can have several levels (e.g. error message when saving a ticket).